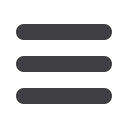

accounts/authentication.py.
import
requests
import
sys
from
accounts.models
import
ListUser
class
PersonaAuthenticationBackend
(
object
):
def
authenticate
(
self
,
assertion
):
# Send the assertion to Mozilla's verifier service.
data
=
{
'assertion'
:
assertion
,
'audience'
:
'localhost'
}
(
'sending to mozilla'
,
data
,
file
=
sys
.
stderr
)
resp
=
requests
.
post
(
'https://verifier.login.persona.org/verify'
,
data
=
data
)
(
'got'
,
resp
.
content
,
file
=
sys
.
stderr
)
# Did the verifier respond?
if
resp
.
ok
:
# Parse the response
verification_data
=
resp
.
json
()
# Check if the assertion was valid
if
verification_data
[
'status'
]
==
'okay'
:
=
verification_data
[
'email'
]
try
:
return
self
.
get_user
(
)
except
ListUser
.
DoesNotExist
:
return
ListUser
.
objects
.
create
(
=
)
def
get_user
(
self
,
):
return
ListUser
.
objects
.
get
(
=
)
This code is copy-pasted directly from the Mozilla site, as you can see from the ex‐
planatory comments.
You’ll need to
pip install requests
into your virtualenv. If you’ve never used it before,
Requestsis a great alternative to the Python standard library tools for HTTP requests.
To finish off the job of customising authentication in Django, we just need a custom
user model:
accounts/models.py.
from
django.contrib.auth.models
import
AbstractBaseUser
,
PermissionsMixin
from
django.db
import
models
class
ListUser
(
AbstractBaseUser
,
PermissionsMixin
):
=
models
.
EmailField
(
primary_key
=
True
)
USERNAME_FIELD
=
'email'
#REQUIRED_FIELDS = ['email', 'height']
objects
=
ListUserManager
()
@property
def
is_staff
(
self
):
return
self
.
==
'harry.percival@example.com'
@property
246
|
Chapter 15: User Authentication, Integrating Third-Party Plugins, and Mocking with JavaScript