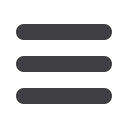

Using Hierarchical Logging Config
When we hacked in the
logging.warning
earlier, we were using the root logger. That’s
not normally a good idea, since any third-party module can mess with the root logger.
The normal pattern is to use a logger named after the file you’re in, by using:
logger
=
logging
.
getLogger
(
__name__
)
Logging configuration is hierarchical, so you can define “parent” loggers for top-level
modules, and all the Python modules inside them will inherit that config.
Here’s how we add a logger for both our apps into
settings.py
:
superlists/settings.py.
LOGGING
=
{
'version'
:
1
,
'disable_existing_loggers'
:
False
,
'handlers'
: {
'console'
: {
'level'
:
'DEBUG'
,
'class'
:
'logging.StreamHandler'
,
},
},
'loggers'
: {
'django'
: {
'handlers'
: [
'console'
],
},
'accounts'
: {
'handlers'
: [
'console'
],
},
'lists'
: {
'handlers'
: [
'console'
],
},
},
'root'
: {
'level'
:
'INFO'
},
}
Now
accounts.models
,
accounts.views
,
accounts.authentication
, and all the others will
inherit the
logging.StreamHandler
from the parent
accounts
logger.
Unfortunately, because of Django’s project structure, there’s no way of defining a top-
level logger for your whole project (aside from using the root logger), so you have to
define one logger per app.
Here’s how to write a test for logging behaviour:
accounts/tests/test_authentication.py (ch17l023).
import
logging
[
...
]
@patch
(
'accounts.authentication.requests.post'
)
class
AuthenticateTest
(
TestCase
):
[
...
]
318
|
Chapter 17: Test Fixtures, Logging, and Server-Side Debugging