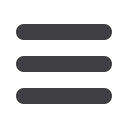

If you’re going to keep any intermediate-level tests at all, I like these three because they
feel like they’re doing themost “integration” jobs: they test the full stack, fromthe request
down to the actual database, and they cover the three most important use cases of our
view.
Conclusions: When to Write Isolated Versus Integrated
Tests
Django’s testing tools make it very easy to quickly put together integrated tests. The test
runner helpfully creates a fast, in-memory version of your database and resets it for you
in between each tests. The
TestCase
class and the Test Client make it easy to test your
views, from checking whether database objects are modified, confirming that your URL
mappings work, and inspecting the rendering of the templates. This lets you get started
with testing very easily and get good coverage across your whole stack.
On the other hand, these kinds of integrated testswon’t necessarily deliver the full benefit
that rigorous unit testing and outside-in TDD are meant to confer in terms of design.
If we look at the example in this chapter, compare the code we had before and after:
Before.
def
new_list
(
request
):
form
=
ItemForm
(
data
=
request
.
POST
)
if
form
.
is_valid
():
list_
=
List
()
if
not
isinstance
(
request
.
user
,
AnonymousUser
):
list_
.
owner
=
request
.
user
list_
.
save
()
form
.
save
(
for_list
=
list_
)
return
redirect
(
list_
)
else
:
return
render
(
request
,
'home.html'
, {
"form"
:
form
})
After.
def
new_list
(
request
):
form
=
NewListForm
(
data
=
request
.
POST
)
if
form
.
is_valid
():
list_
=
form
.
save
(
owner
=
request
.
user
)
return
redirect
(
list_
)
return
render
(
request
,
'home.html'
, {
'form'
:
form
})
If we hadn’t bothered to go down the isolation route, would we have bothered to refactor
the view function? I know I didn’t in the first draft of this book. I’d like to think I would
have “in real life”, but it’s hard to be sure. But writing isolated tests does make you very
aware of where the complexities in your code lie.
362
|
Chapter 19: Test Isolation, and “Listening to Your Tests”