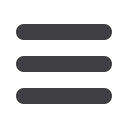

We’ve seen that Selenium provides
WebdriverWait
as a tool for do‐
ing waits, but it’s a little restrictive. This hand-rolled version lets us
pass a function that does a
unittest
assertion, with all the benefits
of the readable error messages that it gives us.
I’ve added the timeout there as an optional argument, and I’m basing it on a constant
we’ll add to
base.py
. We’ll also use it in our original
implicitly_wait
:
functional_tests/base.py (ch20l014).
[
...
]
DEFAULT_WAIT
=
5
SCREEN_DUMP_LOCATION
=
os
.
path
.
abspath
(
os
.
path
.
join
(
os
.
path
.
dirname
(
__file__
),
'screendumps'
)
)
class
FunctionalTest
(
StaticLiveServerCase
):
[
...
]
def
setUp
(
self
):
self
.
browser
=
webdriver
.
Firefox
()
self
.
browser
.
implicitly_wait
(
DEFAULT_WAIT
)
Now we can rerun the test to confirm it still works locally:
$
python3 manage.py test functional_tests.test_my_lists
[...]
.
Ran 1 test in 9.594s
OK
And, just to be sure, we’ll deliberately break our test to see it fail too:
functional_tests/test_my_lists.py (ch20l015).
self
.
wait_for
(
lambda
:
self
.
assertEqual
(
self
.
browser
.
current_url
,
'barf'
)
)
Sure enough, that gives:
$
python3 manage.py test functional_tests.test_my_lists
[...]
AssertionError:
'http://localhost:8081/lists/1/' != 'barf'
And we see it pause on the page for three seconds. Let’s revert that last change, and then
commit our changes:
$
git diff
# base.py, test_my_lists.py
$
git commit -am"use wait_for function for URL checks in my_lists"
$
git push
380
|
Chapter 20: Continuous Integration (CI)