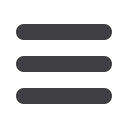

list_page
.
wait_for_new_item_in_list
(
item_text
,
1
)
#
return
list_page
#
It’s initialised with an object that represents the current test. That gives us the
ability to make assertions, access the browser instance via
self.test.brows
er
, and use the
wait_for
function.
Most Page objects have a “go to this page” function. Notice that it implements
the interaction/wait pattern—first we
get
the page URL, then we wait for an
element that we know is on the home page.
Returning
self
is just a convenience. It enables
method chaining .Here’s our function that starts a new list. It goes to the home page, finds the input
box, and sends the new item text to it, as well as a carriage return. Then it uses
a wait to check that the interaction has completed, but as you can see that wait
is actually on a different Page object:
The
ListPage
, which we’ll see the code for shortly. It’s initialised just like a
HomePage
.
We use the
ListPage
to
wait_for_new_item_in_list
. We specify the expected
text of the item, and its expected position in the list.
Finally, we return the
list_page
object to the caller, because they will probably
find it useful (as we’ll see shortly).
Here’s how
ListPage
looks:
functional_tests/home_and_list_pages.py (ch21l006).
[
...
]
class
ListPage
(
object
):
def
__init__
(
self
,
test
):
self
.
test
=
test
def
get_list_table_rows
(
self
):
return
self
.
test
.
browser
.
find_elements_by_css_selector
(
'#id_list_table tr'
)
def
wait_for_new_item_in_list
(
self
,
item_text
,
position
):
expected_row
=
'{}: {}'
.
format
(
position
,
item_text
)
self
.
test
.
wait_for
(
lambda
:
self
.
test
.
assertIn
(
expected_row
,
[
row
.
text
for
row
in
self
.
get_list_table_rows
()]
))
The Page Pattern
|
391