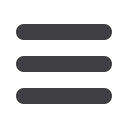

$
git diff
# should show changes to urls.py, tests.py, and views.py
$
git commit -am"First unit test and url mapping, dummy view"
That was the last variation on
git commit
I’ll show, the
a
and
m
flags together, which
adds all changes to tracked files and uses the commit message from the command line.
git commit -am
is the quickest formulation, but also gives you the
least feedback about what’s being committed, so make sure you’ve
done a
git status
and a
git diff
beforehand, and are clear on
what changes are about to go in.
Unit Testing a View
On to writing a test for our view, so that it can be something more than a do-nothing
function, and instead be a function that returns a real response with HTML to the
browser. Open up
lists/tests.py
, and add a new
test method
. I’ll explain each bit:
lists/tests.py.
from
django.core.urlresolvers
import
resolve
from
django.test
import
TestCase
from
django.http
import
HttpRequest
from
lists.views
import
home_page
class
HomePageTest
(
TestCase
):
def
test_root_url_resolves_to_home_page_view
(
self
):
found
=
resolve
(
'/'
)
self
.
assertEqual
(
found
.
func
,
home_page
)
def
test_home_page_returns_correct_html
(
self
):
request
=
HttpRequest
()
#
response
=
home_page
(
request
)
#
self
.
assertTrue
(
response
.
content
.
startswith
(
b
'<html>'
))
#
self
.
assertIn
(
b
'<title>To-Do lists</title>'
,
response
.
content
)
#
self
.
assertTrue
(
response
.
content
.
endswith
(
b
'</html>'
))
#
What’s going on in this new test?
We create an
HttpRequest
object, which is what Django will see when a user’s
browser asks for a page.
We pass it to our
home_page
view, which gives us a response. You won’t be
surprised to hear that this object is an instance of a class called
HttpResponse
.
Then, we assert that the
.content
of the response—which is the HTML that we
send to the user—has certain properties.
30
|
Chapter 3: Testing a Simple Home Page with Unit Tests