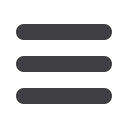

I wanted to show you how to “roll your own” first, partially because it’s a better intro‐
duction to howDjango works, but also because those techniques are portable—youmay
not always use Django, but you’ll almost always have view functions, templates, and
URL mappings, and now you know how to test them.
A New Test Class
So let’s use the Django test client. Open up
lists/tests.py
, and add a new test class called
ListViewTest
. Then copy the method called
test_home_page_displays_all_
list_items
across from
HomePageTest
into our new class, rename it, and adapt it
slightly:
lists/tests.py (ch06l009).
class
ListViewTest
(
TestCase
):
def
test_displays_all_items
(
self
):
Item
.
objects
.
create
(
text
=
'itemey 1'
)
Item
.
objects
.
create
(
text
=
'itemey 2'
)
response
=
self
.
client
.
get
(
'/lists/the-only-list-in-the-world/'
)
#
self
.
assertContains
(
response
,
'itemey 1'
)
#
self
.
assertContains
(
response
,
'itemey 2'
)
#
Instead of calling the view function directly, we use the Django test client, which
is an attribute of the Django
TestCase
called
self.client
. We tell it to
.get
the
URL we’re testing—it’s actually a very similar API to the one that Selenium uses.
Instead of using the slightly annoying
assertIn
/
response.content.decode()
dance, Django provides the
assertContains
method which knows how to deal
with responses and the bytes of their content.
Some people really don’t like the Django test client. They say it pro‐
vides too much magic, and involves too much of the stack to be used
in a real “unit” test—you end up writing what are more properly called
integrated tests. They also complain that it is relatively slow (and
relatively is measured in milliseconds). We’ll explore this argument
further in a later chapter. For now we’ll use it because it’s extremely
convenient!
Let’s try running the test now:
AssertionError: 404 != 200 : Couldn't retrieve content: Response code was 404
A New URL
Our singleton list URL doesn’t exist yet. We fix that in
superlists/urls.py
.
88
|
Chapter 6: Getting to the Minimum Viable Site