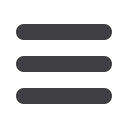

lists/views.py.
def
new_list
(
request
):
return
redirect
(
'/lists/the-only-list-in-the-world/'
)
That gives:
self.assertEqual(Item.objects.count(), 1)
AssertionError: 0 != 1
[...]
AssertionError:
'http://testserver/lists/the-only-list-in-the-world/'!=
'/lists/the-only-list-in-the-world/'
Let’s start with the first failure, because it’s reasonably straightforward. We borrow an‐
other line from
home_page
:
lists/views.py.
def
new_list
(
request
):
Item
.
objects
.
create
(
text
=
request
.
POST
[
'item_text'
])
return
redirect
(
'/lists/the-only-list-in-the-world/'
)
And that takes us down to just the second, unexpected failure:
self.assertEqual(response['location'],
'/lists/the-only-list-in-the-world/')
AssertionError:
'http://testserver/lists/the-only-list-in-the-world/'!=
'/lists/the-only-list-in-the-world/'
It’s happening because the Django test client behaves slightly differently to our pure view
function; it’s using the full Django stack which adds the domain to our relative URL.
Let’s use another of Django’s test helper functions, instead of our two-step check for the
redirect:
lists/tests.py.
def
test_redirects_after_POST
(
self
):
response
=
self
.
client
.
post
(
'/lists/new'
,
data
=
{
'item_text'
:
'A new list item'
}
)
self
.
assertRedirects
(
response
,
'/lists/the-only-list-in-the-world/'
)
That now passes:
Ran 8 tests in 0.030s
OK
Removing Now-Redundant Code and Tests
We’re looking good. Since our newviews are nowdoingmost of the work that
home_page
used to do, we should be able to massively simplify it. Can we remove the whole
if
request.method == 'POST'
section, for example?
Another URL and View for Adding List Items
|
95