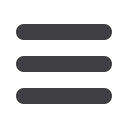

+ saved_list = List.objects.first()
+ self.assertEqual(saved_list, list_)
+
saved_items = Item.objects.all()
self.assertEqual(saved_items.count(), 2)
first_saved_item = saved_items[0]
second_saved_item = saved_items[1]
self.assertEqual(first_saved_item.text, 'The first (ever) list item')
+ self.assertEqual(first_saved_item.list, list_)
self.assertEqual(second_saved_item.text, 'Item the second')
+ self.assertEqual(second_saved_item.list, list_)
We create a new
List
object, and then we assign each item to it by assigning it as
its
.list
property. We check the list is properly saved, and we check that the two items
have also saved their relationship to the list. You’ll also notice that we can compare list
objects with each other directly (
saved_list
and
list
)—behind the scenes, these will
compare themselves by checking their primary key (the
.id
attribute) is the same.
I’m using the variable name
list_
to avoid “shadowing” the Python
built-in
list
function. It’s ugly, but all the other options I tried were
equally ugly or worse (
my_list
,
the_list
,
list1
,
listey
…).
Time for another unit-test/code cycle.
For the first couple of iterations, rather than explicitly showing you what code to enter
in between every test run, I’m only going to show you the expected error messages from
running the tests. I’ll let you figure out what each minimal code change should be on
your own:
Your first error should be:
ImportError: cannot import name 'List'
Fix that, then you should see:
AttributeError: 'List' object has no attribute 'save'
Next you should see:
django.db.utils.OperationalError: no such table: lists_list
So we run a
makemigrations
:
$
python3 manage.py makemigrations
Migrations for 'lists':
0003_list.py:
- Create model List
And then you should see:
98
|
Chapter 6: Getting to the Minimum Viable Site