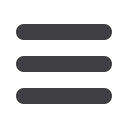

1. If you’re using Python 3.2, upgrade! Or if you’re stuck with it,
pip3 install mock
, and use
from mock
instead of
from unittest.mock
.
Mocking in Python
The popular
mock
package was added to the standard library as part of Python 3.3.
1
It
provides a magical object called a
Mock
, which is a bit like the Sinon mock objects we
saw in the last chapter, only much cooler. Check this out:
>>> from unittest.mock import Mock
>>> m = Mock()
>>> m.any_attribute
<Mock name='mock.any_attribute' id='140716305179152'>
>>> m.foo
<Mock name='mock.foo' id='140716297764112'>
>>> m.any_method()
<Mock name='mock.any_method()' id='140716331211856'>
>>> m.foo()
<Mock name='mock.foo()' id='140716331251600'>
>>> m.called
False
>>> m.foo.called
True
>>> m.bar.return_value = 1
>>> m.bar()
1
A mock object would be a pretty neat thing to use to mock out the
authenticate
function, wouldn’t it? Here’s how you can do that.
Testing Our View by Mocking Out authenticate
(I trust you to set up a tests folder with a dunderinit. Don’t forget to delete the default
tests.py
, as well.)
accounts/tests/test_views.py.
from
django.test
import
TestCase
from
unittest.mock
import
patch
class
LoginViewTest
(
TestCase
):
@patch
(
'accounts.views.authenticate'
)
#
def
test_calls_authenticate_with_assertion_from_post
(
self
,
mock_authenticate
#
):
mock_authenticate
.
return_value
=
None
#
self
.
client
.
post
(
'/accounts/login'
, {
'assertion'
:
'assert this'
})
mock_authenticate
.
assert_called_once_with
(
assertion
=
'assert this'
)
#
278
|
Chapter 16: Server-Side Authentication and Mocking in Python