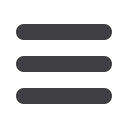

Checking the View Actually Logs the User In
But our
authenticate
view also needs to actually log the user in by calling the Django
auth.login
function, if
authenticate
returns a user. Then it needs to return something
other than an empty response—since this is an Ajax view, it doesn’t need to return
HTML, just an “OK” string will do:
accounts/tests/test_views.py (ch16l011).
from
django.contrib.auth
import
get_user_model
from
django.test
import
TestCase
from
unittest.mock
import
patch
User
=
get_user_model
()
#
class
LoginViewTest
(
TestCase
):
@patch
(
'accounts.views.authenticate'
)
def
test_calls_authenticate_with_assertion_from_post
(
[
...
]
@patch
(
'accounts.views.authenticate'
)
def
test_returns_OK_when_user_found
(
self
,
mock_authenticate
):
user
=
User
.
objects
.
create
(
=
'a@b.com'
)
user
.
backend
=
''
# required for auth_login to work
mock_authenticate
.
return_value
=
user
response
=
self
.
client
.
post
(
'/accounts/login'
, {
'assertion'
:
'a'
})
self
.
assertEqual
(
response
.
content
.
decode
(),
'OK'
)
I should explain this use of
get_user_model
from
django.contrib.auth
. Its job
is to find the project’s user model, and it works whether you’re using the standard
user model or a custom one (like we will be).
That test covers the desired response. Now test that the user actually gets logged in
correctly. We can do that by inspecting the Django test client, to see if the session cookie
has been set correctly.
Check out the
Django docs on authenticationat this point.
accounts/tests/test_views.py (ch16l012).
from
django.contrib.auth
import
get_user_model
,
SESSION_KEY
[
...
]
@patch
(
'accounts.views.authenticate'
)
def
test_gets_logged_in_session_if_authenticate_returns_a_user
(
self
,
mock_authenticate
Mocking in Python
|
281