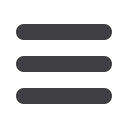

mock_user
=
mock_authenticate
.
return_value
login
(
request
)
mock_login
.
assert_called_once_with
(
request
,
mock_user
)
The upside of this version of the test is that it doesn’t need to rely on the magic of the
Django test client, and it doesn’t need to know anything about how Django sessions
work—all you need to know is the name of the function you’re supposed to call.
Its downside is that it is verymuch testing implementation, rather than testing behaviour
—it’s tightly coupled to the particular name of the Django login function and its API.
De-spiking Our Custom Authentication Backend: Mocking
Out an Internet Request
Our custom authentication backend is next. Here’s how it looked in the spike:
class
PersonaAuthenticationBackend
(
object
):
def
authenticate
(
self
,
assertion
):
# Send the assertion to Mozilla's verifier service.
data
=
{
'assertion'
:
assertion
,
'audience'
:
'localhost'
}
(
'sending to mozilla'
,
data
,
file
=
sys
.
stderr
)
resp
=
requests
.
post
(
'https://verifier.login.persona.org/verify'
,
data
=
data
)
(
'got'
,
resp
.
content
,
file
=
sys
.
stderr
)
# Did the verifier respond?
if
resp
.
ok
:
# Parse the response
verification_data
=
resp
.
json
()
# Check if the assertion was valid
if
verification_data
[
'status'
]
==
'okay'
:
=
verification_data
[
'email'
]
try
:
return
self
.
get_user
(
)
except
ListUser
.
DoesNotExist
:
return
ListUser
.
objects
.
create
(
=
)
def
get_user
(
self
,
):
return
ListUser
.
objects
.
get
(
=
)
Decoding this:
• We take an assertion and send it off to Mozilla using
requests.post
.
• We check its response code (
resp.ok
), and then check for a
status=okay
in the
response JSON.
• We then extract an email address, and either find an existing user with that address,
or create a new one.
De-spiking Our Custom Authentication Backend: Mocking Out an Internet Request
|
285