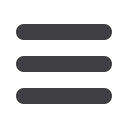

mock_post
.
return_value
.
ok
=
False
#
user
=
self
.
backend
.
authenticate
(
'an assertion'
)
self
.
assertIsNone
(
user
)
def
test_returns_none_if_status_not_okay
(
self
,
mock_post
):
mock_post
.
return_value
.
json
.
return_value
=
{
'status'
:
'not okay!'
}
#
user
=
self
.
backend
.
authenticate
(
'an assertion'
)
self
.
assertIsNone
(
user
)
You can apply a
patch
at the class level as well, and that has the effect that every
test method in the class will have the patch applied, and the mock injected.
We can now use the
setUp
function to prepare any useful variables which we’re
going to use in all of our tests.
Now each test is only adjusting the setup variables
it
needs, rather than setting
up a load of duplicated boilerplate—it’s more readable.
And that’s all very well, but everything still passes!
OK
Time to test for the positive case where
authenticate
should return a user object. We
expect this to fail:
accounts/tests/test_authentication.py (ch16l022-1).
from
django.contrib.auth
import
get_user_model
User
=
get_user_model
()
[
...
]
def
test_finds_existing_user_with_email
(
self
,
mock_post
):
mock_post
.
return_value
.
json
.
return_value
=
{
'status'
:
'okay'
,
'email'
:
'a@b.com'}
actual_user
=
User
.
objects
.
create
(
=
'a@b.com')
found_user
=
self
.
backend
.
authenticate
(
'an assertion'
)
self
.
assertEqual
(
found_user
,
actual_user
)
Indeed, a fail:
AssertionError: None != <User: >
Let’s code. We’ll start with a “cheating” implementation, where we just get the first user
we find in the database:
accounts/authentication.py (ch16l023).
import
requests
from
django.contrib.auth
import
get_user_model
User
=
get_user_model
()
[
...
]
def
authenticate
(
self
,
assertion
):
requests
.
post
(
PERSONA_VERIFY_URL
,
data
=
{
'assertion'
:
assertion
,
'audience'
:
DOMAIN
}
)
return
User
.
objects
.
first
()
288
|
Chapter 16: Server-Side Authentication and Mocking in Python