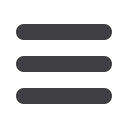

Here’s a couple of tests for those two requirements:
accounts/tests/test_authentication.py (ch16l030).
class
GetUserTest
(
TestCase
):
def
test_gets_user_by_email
(
self
):
backend
=
PersonaAuthenticationBackend
()
other_user
=
User
(
=
'other@user.com'
)
other_user
.
username
=
'otheruser'
other_user
.
save
()
desired_user
=
User
.
objects
.
create
(
=
'a@b.com'
)
found_user
=
backend
.
get_user
(
'a@b.com'
)
self
.
assertEqual
(
found_user
,
desired_user
)
def
test_returns_none_if_no_user_with_that_email
(
self
):
backend
=
PersonaAuthenticationBackend
()
self
.
assertIsNone
(
backend
.
get_user
(
'a@b.com'
)
)
Here’s our first failure:
AttributeError: 'PersonaAuthenticationBackend' object has no attribute
'get_user'
Let’s create a placeholder one then:
accounts/authentication.py (ch16l031).
class
PersonaAuthenticationBackend
(
object
):
def
authenticate
(
self
,
assertion
):
[
...
]
def
get_user
(
self
):
pass
Now we get:
TypeError: get_user() takes 1 positional argument but 2 were given
So:
accounts/authentication.py (ch16l032).
def
get_user
(
self
,
):
pass
Next:
self.assertEqual(found_user, desired_user)
AssertionError: None != <User: >
And (step by step, just to see if our test fails the way we think it will):
accounts/authentication.py (ch16l033).
def
get_user
(
self
,
):
return
User
.
objects
.
first
()
292
|
Chapter 16: Server-Side Authentication and Mocking in Python