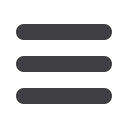

That works:
$
python3 manage.py test accounts
[...]
Ran 13 tests in 0.021s
OK
One final cleanup of migrations to make sure we’ve got everything there:
$
rm accounts/migrations/0001_initial.py
$
python3 manage.py makemigrations
Migrations for 'accounts':
0001_initial.py:
- Create model User
No warnings now!
Users Are Authenticated
Our usermodel needs one last property before it’s complete: standardDjango users have
an API which includes
several methods ,most of which we won’t need, but there is one
that will come in useful:
.is_authenticated()
:
accounts/tests/test_models.py (ch16l045).
def
test_is_authenticated
(
self
):
user
=
User
()
self
.
assertTrue
(
user
.
is_authenticated
())
Which gives:
AttributeError: 'User' object has no attribute 'is_authenticated'
And so, the ultra-simple:
accounts/models.py.
class
User
(
models
.
Model
):
=
models
.
EmailField
(
primary_key
=
True
)
last_login
=
models
.
DateTimeField
(
default
=
timezone
.
now
)
REQUIRED_FIELDS
=
()
USERNAME_FIELD
=
'email'
def
is_authenticated
(
self
):
return
True
And that works:
$
python3 manage.py test accounts
[...]
Ran 14 tests in 0.021s
OK
A Minimal Custom User Model
|
297