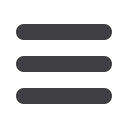

4. You might ask, if I think Django is so silly, why don’t I submit a pull request to fix it? Should be quite a simple
fix. Well, I promise I will, as soon as I’ve finished writing the book. For now, snarky comments will have to
suffice.
accounts/tests/test_models.py.
from
django.test
import
TestCase
from
django.contrib.auth
import
get_user_model
User
=
get_user_model
()
class
UserModelTest
(
TestCase
):
def
test_user_is_valid_with_email_only
(
self
):
user
=
User
(
=
'a@b.com'
)
user
.
full_clean
()
# should not raise
That gives us an expected failure:
django.core.exceptions.ValidationError: {'username': ['This field cannot be
blank.'], 'password': ['This field cannot be blank.']}
Password? Username? Bah! How about this?
accounts/models.py.
from
django.db
import
models
class
User
(
models
.
Model
):
=
models
.
EmailField
()
And we wire it up inside
settings.py
using a variable called
AUTH_USER_MODEL
. While
we’re at it, we’ll add our new authentication backend too:
superlists/settings.py (ch16l039).
AUTH_USER_MODEL
=
'accounts.User'
AUTHENTICATION_BACKENDS
=
(
'accounts.authentication.PersonaAuthenticationBackend'
,
)
Now Django tells us off because it wants a couple of bits of metadata on any custom
user model:
AttributeError: type object 'User' has no attribute 'REQUIRED_FIELDS'
Sigh. Come on, Django, it’s only got one field, you should be able to figure out the
answers to these questions for yourself. Here you go:
accounts/models.py.
class
User
(
models
.
Model
):
=
models
.
EmailField
()
REQUIRED_FIELDS
=
()
Next silly question?
4
AttributeError: type object 'User' has no attribute 'USERNAME_FIELD'
So:
294
|
Chapter 16: Server-Side Authentication and Mocking in Python