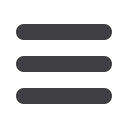

I also found that improving the failure message in the
wait_for_element_with_id
function helped to see what was going on:
functional_tests/test_login.py.
def
wait_for_element_with_id
(
self
,
element_id
):
WebDriverWait
(
self
.
browser
,
timeout
=
30
)
.
until
(
lambda
b
:
b
.
find_element_by_id
(
element_id
),
'Could not find element with id {}. Page text was {}'
.
format
(
element_id
,
self
.
browser
.
find_element_by_tag_name
(
'body'
)
.
text
)
)
With that, we can see that the test is failing because the logout button doesn’t work:
$
python3 manage.py test functional_tests.test_login
File "/workspace/superlists/functional_tests/test_login.py", line 36, in
wait_to_be_logged_out
[...]
selenium.common.exceptions.TimeoutException: Message: 'Could not find element
with id id_login. Page text was Superlists\nLog out\nLogged in as
edith@mockmyid.com \nStart a new To-Do list'
Implementing a logout button is actually very simple: we canuseDjango’s
built-in logout view ,which clears down the user’s session and redirects them to a page of our choice:
accounts/urls.py.
urlpatterns
=
patterns
(
''
,
url
(
r'^login$'
,
'accounts.views.persona_login'
,
name
=
'persona_login'
),
url
(
r'^logout$'
,
'django.contrib.auth.views.logout'
, {
'next_page'
:
'/'
},
name
=
'logout'
),
)
And in
base.html
, we just make the logout into a normal URL link:
lists/templates/base.html.
<
a
class
=
"btn navbar-btn navbar-right"
id
=
"id_logout"
href
=
"{
% u
rl 'logout' %}"
>
Log
out
</
a
>
And that gets us a fully passing FT—indeed, a fully passing test suite:
$
python3 manage.py test functional_tests.test_login
[...]
OK
$
python3 manage.py test
[...]
Ran 54 tests in 78.124s
OK
In the next chapter, we’ll start trying to put our login system to good use. In the mean‐
time, do a commit, and enjoy this recap:
300
|
Chapter 16: Server-Side Authentication and Mocking in Python