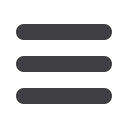

OK
The Next “Requirement” from the Views Layer: New Lists
Should Record Owner
Before we move down to the model layer, there’s another part of the code at the views
layer that will need to use our model: we need some way for newly created lists to be
assigned to an owner, if the current user is logged in to the site.
Here’s a first crack at writing the test:
lists/tests/test_views.py (ch18l014).
from
django.http
import
HttpRequest
[
...
]
from
lists.views
import
new_list
[
...
]
class
NewListTest
(
TestCase
):
[
...
]
def
test_list_owner_is_saved_if_user_is_authenticated
(
self
):
request
=
HttpRequest
()
request
.
user
=
User
.
objects
.
create
(
=
'a@b.com'
)
request
.
POST
[
'text'
]
=
'new list item'
new_list
(
request
)
list_
=
List
.
objects
.
first
()
self
.
assertEqual
(
list_
.
owner
,
request
.
user
)
This test uses the raw view function, and manually constructs an
HttpRequest
because
it’s slightly easier to write the test that way. Although the Django test client does have a
helper function called
login
, it doesn’t work well with external authentication services.
The alternative would be tomanually create a session object (like we do in the functional
tests), or to use mocks, and I think both of those would end up uglier than this version.
If you’re curious, you could have a go at writing it differently.
The test fails as follows:
AttributeError: 'List' object has no attribute 'owner'
To fix this, we can try writing code like this:
lists/views.py (ch18l015).
def
new_list
(
request
):
form
=
ItemForm
(
data
=
request
.
POST
)
if
form
.
is_valid
():
list_
=
List
()
list_
.
owner
=
request
.
user
list_
.
save
()
form
.
save
(
for_list
=
list_
)
return
redirect
(
list_
)
330
|
Chapter 18: Finishing “My Lists”: Outside-In TDD