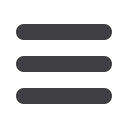

def
test_get_absolute_url
(
self
):
[
...
]
def
test_lists_can_have_owners
(
self
):
user
=
User
.
objects
.
create
(
=
'
a@b.com'
)
list_
=
List
.
objects
.
create
(
owner
=
user
)
self
.
assertIn
(
list_
,
user
.
list_set
.
all
())
And that gives us a new unit test failure:
list_ = List.objects.create(owner=user)
[...]
TypeError: 'owner' is an invalid keyword argument for this function
The naive implementation would be this:
from
django.conf
import
settings
[
...
]
class
List
(
models
.
Model
):
owner
=
models
.
ForeignKey
(
settings
.
AUTH_USER_MODEL
)
But we want to make sure the list owner is optional. Explicit is better than implicit, and
tests are documentation, so let’s have a test for that too:
lists/tests/test_models.py (ch18l020).
def
test_list_owner_is_optional
(
self
):
List
.
objects
.
create
()
# should not raise
The correct implementation is this:
lists/models.py.
from
django.conf
import
settings
[
...
]
class
List
(
models
.
Model
):
owner
=
models
.
ForeignKey
(
settings
.
AUTH_USER_MODEL
,
blank
=
True
,
null
=
True
)
def
get_absolute_url
(
self
):
return
reverse
(
'view_list'
,
args
=
[
self
.
id
])
Now running the tests gives the usual database error:
return Database.Cursor.execute(self, query, params)
django.db.utils.OperationalError: table lists_list has no column named owner_id
Because we need to do make some migrations:
$
python3 manage.py makemigrations
Migrations for 'lists':
0006_list_owner.py:
- Add field owner to list
We’re almost there, a couple more failures:
ERROR: test_redirects_after_POST (lists.tests.test_views.NewListTest)
[...]
332
|
Chapter 18: Finishing “My Lists”: Outside-In TDD