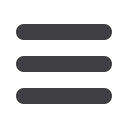

lists/tests/test_views.py.
class
NewListTest
(
TestCase
):
[
...
]
def
test_list_owner_is_saved_if_user_is_authenticated
(
self
):
request
=
HttpRequest
()
request
.
user
=
User
.
objects
.
create
(
=
'a@b.com'
)
request
.
POST
[
'text'
]
=
'new list item'
new_list
(
request
)
list_
=
List
.
objects
.
first
()
self
.
assertEqual
(
list_
.
owner
,
request
.
user
)
And here’s what our attempted solution looked like:
lists/views.py.
def
new_list
(
request
):
form
=
ItemForm
(
data
=
request
.
POST
)
if
form
.
is_valid
():
list_
=
List
()
list_
.
owner
=
request
.
user
list_
.
save
()
form
.
save
(
for_list
=
list_
)
return
redirect
(
list_
)
else
:
return
render
(
request
,
'home.html'
, {
"form"
:
form
})
And at this point, the view test is failing because we don’t have the model layer yet:
self.assertEqual(list_.owner, request.user)
AttributeError: 'List' object has no attribute 'owner'
You won’t see this error unless you actually check out the old code
and revert
lists/models.py
. You should definitely do this, part of the
objective of this chapter is to see whether we really can write tests for
a models layer that doesn’t exist yet.
A First Attempt at Using Mocks for Isolation
Lists don’t have owners yet, but we can let the views layer tests pretend they do by using
a bit of mocking:
lists/tests/test_views.py (ch19l003).
from
unittest.mock
import
Mock
,
patch
from
django.http
import
HttpRequest
from
django.test
import
TestCase
[
...
]
@patch
(
'lists.views.List'
)
#
def
test_list_owner_is_saved_if_user_is_authenticated
(
self
,
mockList
):
mock_list
=
List
.
objects
.
create
()
#
mock_list
.
save
=
Mock
()
338
|
Chapter 19: Test Isolation, and “Listening to Your Tests”