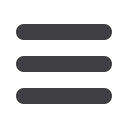

A New Test Suite with Full Isolation
Let’s start with a blank slate, and see if we can use isolated tests to drive a replacement
of our
new_list
view. We’ll call it
new_list2
, build it alongside the old view, and when
we’re ready, we can swap it in and see if the old integrated tests all still pass.
lists/views.py (ch19l009).
def
new_list
(
request
):
[
...
]
def
new_list2
(
request
):
pass
Thinking in Terms of Collaborators
In order to rewrite our tests to be fully isolated, we need to throw out our old way of
thinking about the tests in terms of the “real” effects of the view on things like the
database, and instead think of it in terms of the objects it collaborates with, and how it
interacts with them.
In the new world, the view’s main collaborator will be a form object, so we mock that
out in order to be able to fully control it, and in order to be able to define, by wishful
thinking, the way we want our form to work.
lists/tests/test_views.py (ch19l010).
from
lists.views
import
new_list
,
new_list2
[
...
]
@patch
(
'lists.views.NewListForm'
)
#
class
NewListViewUnitTest
(
unittest
.
TestCase
):
#
def
setUp
(
self
):
self
.
request
=
HttpRequest
()
self
.
request
.
POST
[
'text'
]
=
'new list item'
#
def
test_passes_POST_data_to_NewListForm
(
self
,
mockNewListForm
):
new_list2
(
self
.
request
)
mockNewListForm
.
assert_called_once_with
(
data
=
self
.
request
.
POST
)
#
The Django
TestCase
class makes it too easy to write integrated tests. As a way
of making sure we’re writing “pure”, isolated unit tests, we’ll only use
uni
ttest.TestCase
We mock out the NewListForm class (which doesn’t even exist yet). It’s going to
be used in all the tests, so we mock it out at the class level.
We set up a basic POST request in
setUp
, building up the request by hand rather
than using the (overly integrated) Django Test Client.
And we check the first thing about our new view: it initialises its collaborator,
the
NewListForm
, with the correct constructor—the data from the request.
Rewriting Our Tests for the View to Be Fully Isolated
|
343