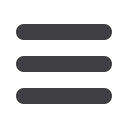

That will start with a failure, saying we don’t have a
NewListForm
in our view yet.
AttributeError: <module 'lists.views' from
'/workspace/superlists/lists/views.py'> does not have the attribute
'NewListForm'
Let’s create a placeholder for it:
lists/views.py (ch19l011).
from
lists.forms
import
ExistingListItemForm
,
ItemForm
,
NewListForm
[
...
]
and:
lists/forms.py (ch19l012).
class
ItemForm
(
forms
.
models
.
ModelForm
):
[
...
]
class
NewListForm
(
object
):
pass
class
ExistingListItemForm
(
ItemForm
):
[
...
]
Next we get a real failure:
AssertionError: Expected 'NewListForm' to be called once. Called 0 times.
And we implement like this:
lists/views.py (ch19l012-2).
def
new_list2
(
request
):
NewListForm
(
data
=
request
.
POST
)
$
python3 manage.py test lists
[...]
Ran 38 tests in 0.143s
OK
Let’s continue. If the form is valid, we want to call save on it:
lists/tests/test_views.py (ch19l013).
@patch
(
'lists.views.NewListForm'
)
class
NewListViewUnitTest
(
unittest
.
TestCase
):
def
setUp
(
self
):
self
.
request
=
HttpRequest
()
self
.
request
.
POST
[
'text'
]
=
'new list item'
self
.
request
.
user
=
Mock
()
def
test_passes_POST_data_to_NewListForm
(
self
,
mockNewListForm
):
new_list2
(
self
.
request
)
mockNewListForm
.
assert_called_once_with
(
data
=
self
.
request
.
POST
)
def
test_saves_form_with_owner_if_form_valid
(
self
,
mockNewListForm
):
344
|
Chapter 19: Test Isolation, and “Listening to Your Tests”