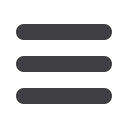

So we actually need to check, not just that the owner is assigned, but that it’s assigned
before
we call save on our list object.
Here’s how we can test the sequence of events using mocks—you can mock out a func‐
tion, and use it as a spy to check on the state of the world at the moment it’s called:
lists/tests/test_views.py (ch19l005).
@patch
(
'lists.views.List'
)
def
test_list_owner_is_saved_if_user_is_authenticated
(
self
,
mockList
):
mock_list
=
List
.
objects
.
create
()
mock_list
.
save
=
Mock
()
mockList
.
return_value
=
mock_list
request
=
HttpRequest
()
request
.
user
=
Mock
()
request
.
user
.
is_authenticated
.
return_value
=
True
request
.
POST
[
'text'
]
=
'new list item'
def
check_owner_assigned
():
#
self
.
assertEqual
(
mock_list
.
owner
,
request
.
user
)
#
mock_list
.
save
.
side_effect
=
check_owner_assigned
#
new_list
(
request
)
mock_list
.
save
.
assert_called_once_with
()
#
We define a function that makes the assertion about the thing we want to happen
first: checking the list’s owner has been set.
We assign that check function as a
side_effect
to the thing we want to check
happened second. When the view calls our mocked save function, it will go
through this assertion. We make sure to set this up before we actually call the
function we’re testing.
Finally, we make sure that the function with the
side_effect
was actually
triggered, ie we did
.save()
. Otherwise our assertion may actually never have
been run.
Two common mistakes when using mock side-effects are: assigning
the side effect too late, i.e.
after
you call the function under test, and
forgetting to check that the side-effect function was actually called.
And by common, I mean, “I made them both several times while
writing this chapter”.
At this point, if you’ve still got the “broken” code from above, where we assign the owner
but call save in the wrong order, you should now see a fail:
ERROR: test_list_owner_is_saved_if_user_is_authenticated
(lists.tests.test_views.NewListTest)
[...]
File "/workspace/superlists/lists/views.py", line 17, in new_list
340
|
Chapter 19: Test Isolation, and “Listening to Your Tests”