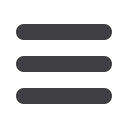

lists/tests/test_forms.py (ch19l021).
import
unittest
from
unittest.mock
import
patch
,
Mock
from
django.test
import
TestCase
from
lists.forms
import
(
DUPLICATE_ITEM_ERROR
,
EMPTY_LIST_ERROR
,
ExistingListItemForm
,
ItemForm
,
NewListForm
)
from
lists.models
import
Item
,
List
[
...
]
class
NewListFormTest
(
unittest
.
TestCase
):
@patch
(
'lists.forms.List.create_new'
)
def
test_save_creates_new_list_from_post_data_if_user_not_authenticated
(
self
,
mock_List_create_new
):
user
=
Mock
(
is_authenticated
=
lambda
:
False
)
form
=
NewListForm
(
data
=
{
'text'
:
'new item text'
})
form
.
is_valid
()
form
.
save
(
owner
=
user
)
mock_List_create_new
.
assert_called_once_with
(
first_item_text
=
'new item text'
)
And while we’re at it we can test the case where the user is an authenticated user too:
lists/tests/test_forms.py (ch19l022).
@patch
(
'lists.forms.List.create_new'
)
def
test_save_creates_new_list_with_owner_if_user_authenticatd
(
self
,
mock_List_create_new
):
user
=
Mock
(
is_authenticated
=
lambda
:
True
)
form
=
NewListForm
(
data
=
{
'text'
:
'new item text'
})
form
.
is_valid
()
form
.
save
(
owner
=
user
)
mock_List_create_new
.
assert_called_once_with
(
first_item_text
=
'new item text'
,
owner
=
user
)
You can see this is a much more readable test. Let’s start implementing our new form.
We start with the import:
lists/forms.py (ch19l023).
from
lists.models
import
Item
,
List
Now mock tells us to create a placeholder for our
create_new
method:
AttributeError: <class 'lists.models.List'> does not have the attribute
'create_new'
lists/models.py.
class
List
(
models
.
Model
):
Moving Down to the Forms Layer
|
349