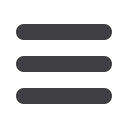

def
test_create_new_optionally_saves_owner
(
self
):
user
=
User
.
objects
.
create
()
List
.
create_new
(
first_item_text
=
'new item text'
,
owner
=
user
)
new_list
=
List
.
objects
.
first
()
self
.
assertEqual
(
new_list
.
owner
,
user
)
And while we’re at it, we can write the tests for the new owner attribute:
lists/tests/test_models.py (ch19l029).
class
ListModelTest
(
TestCase
):
[
...
]
def
test_lists_can_have_owners
(
self
):
List
(
owner
=
User
())
# should not raise
def
test_list_owner_is_optional
(
self
):
List
()
.
full_clean
()
# should not raise
These two are almost exactly the same tests we used in the last chapter, but I’ve re-written
themslightly so they don’t actually save objects—just having themas in-memory objects
is enough to for this test.
Use in-memory (unsaved) model objects in your tests whenever you
can, it makes your tests faster.
That gives:
$
python3 manage.py test lists
[...]
ERROR: test_create_new_optionally_saves_owner
TypeError: create_new() got an unexpected keyword argument 'owner'
[...]
ERROR: test_lists_can_have_owners (lists.tests.test_models.ListModelTest)
TypeError: 'owner' is an invalid keyword argument for this function
[...]
Ran 48 tests in 0.204s
FAILED (errors=2)
We implement, just like we did in the last chapter:
lists/models.py (ch19l030-1).
from
django.conf
import
settings
[
...
]
class
List
(
models
.
Model
):
owner
=
models
.
ForeignKey
(
settings
.
AUTH_USER_MODEL
,
blank
=
True
,
null
=
True
)
[
...
]
352
|
Chapter 19: Test Isolation, and “Listening to Your Tests”