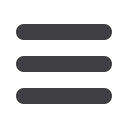

FAILED (failures=5, errors=6)
Not totally obvious, but a bit of Googling around led me to understand that I needed
to use a “named” regex capture group:
lists/urls.py (ch31l011).
@@ -1,7 +1,7 @@
from django.conf.urls import patterns, url
-from lists.views import NewListView
+from lists.views import NewListView, ViewAndAddToList
urlpatterns = patterns('',
- url(r'^(\d+)/$', 'lists.views.view_list', name='view_list'),
+ url(r'^(?P<pk>\d+)/$', ViewAndAddToList.as_view(), name='view_list'),
url(r'^new$', NewListView.as_view(), name='new_list'),
)
The next error was fairly helpful:
[...]
django.template.base.TemplateDoesNotExist: lists/list_detail.html
FAILED (failures=5, errors=6)
That’s easily solved:
lists/views.py.
class
ViewAndAddToList
(
DetailView
):
model
=
List
template_name
=
'list.html'
That takes us down three errors:
[...]
ERROR: test_displays_item_form (lists.tests.test_views.ListViewTest)
KeyError: 'form'
FAILED (failures=5, errors=2)
Until We’re Left with Trial and Error
So I figured, our view doesn’t just show us the detail of an object, it also allows us to
create new ones. Let’s make it both a
DetailView
and
a
CreateView
:
lists/views.py.
class
ViewAndAddToList
(
DetailView
,
CreateView
):
model
=
List
template_name
=
'list.html'
form_class
=
ExistingListItemForm
But that gives us a lot of errors saying:
[...]
TypeError: __init__() missing 1 required positional argument: 'for_list'
418
|
Appendix B: Django Class-Based Views