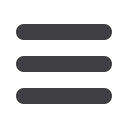

def
test_cbv_gets_correct_object
(
self
):
our_list
=
List
.
objects
.
create
()
view
=
ViewAndAddToList
()
view
.
kwargs
=
dict
(
pk
=
our_list
.
id
)
self
.
assertEqual
(
view
.
get_object
(),
our_list
)
But the problem is that it requires a lot of knowledge of the internals of Django CBVs
to be able to do the right test setup for these kinds of tests. And you still end up getting
very confused by the complex inheritance hierarchy.
Take-Home: Having Multiple, Isolated View Tests with Single
Assertions Helps
One thing I definitely did conclude from this appendix was that having many short unit
tests for views was much more helpful than having few tests with a narrative series of
assertions.
Consider this monolithic test:
def
test_validation_errors_sent_back_to_home_page_template
(
self
):
response
=
self
.
client
.
post
(
'/lists/new'
,
data
=
{
'text'
:
''
})
self
.
assertEqual
(
List
.
objects
.
all
()
.
count
(),
0
)
self
.
assertEqual
(
Item
.
objects
.
all
()
.
count
(),
0
)
self
.
assertTemplateUsed
(
response
,
'home.html'
)
expected_error
=
escape
(
"You can't have an empty list item"
)
self
.
assertContains
(
response
,
expected_error
)
That is definitely less useful than having three individual tests, like this:
def
test_invalid_input_means_nothing_saved_to_db
(
self
):
self
.
post_invalid_input
()
self
.
assertequal
(
item
.
objects
.
all
()
.
count
(),
0
)
def
test_invalid_input_renders_list_template
(
self
):
response
=
self
.
post_invalid_input
()
self
.
asserttemplateused
(
response
,
'list.html'
)
def
test_invalid_input_renders_form_with_errors
(
self
):
response
=
self
.
post_invalid_input
()
self
.
assertisinstance
(
response
.
context
[
'form'
],
existinglistitemform
)
self
.
assertcontains
(
response
,
escape
(
empty_list_error
))
The reason is that, in the first case, an early failure means not all the assertions are
checked. So, if the view was accidentally saving to the database on invalid POST, you
would get an early fail, and so you wouldn’t find out whether it was using the right
template or rendering the form. The second formulation makes it much easier to pick
out exactly what was or wasn’t working.
Best Practices for Unit Testing CBGVs?
|
421