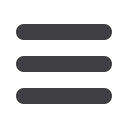

<table
id=
"id_list_table"
>
<tr><td>
{{ new_item_text }}
</td></tr>
</table>
</body>
How can we test that our view is passing in the correct value for
new_item_text
? How
do we pass a variable to a template? We can find out by actually doing it in the unit test
—we’ve already used the
render_to_string
function in a previous unit test tomanually
render a template and compare it with the HTML the view returns. Now let’s add the
variable we want to pass in:
lists/tests.py.
self
.
assertIn
(
'A new list item'
,
response
.
content
.
decode
())
expected_html
=
render_to_string
(
'home.html'
,
{
'new_item_text'
:
'A new list item'
}
)
self
.
assertEqual
(
response
.
content
.
decode
(),
expected_html
)
As you can see, the
render_to_string
function takes, as its second parameter, a map‐
ping of variable names to values. We’re giving the template a variable named
new_item_text
, whose value is the expected item text from our POST request.
When we run the unit test,
render_to_string
will substitute
{{ new_item_text }}
for
A new list item
inside the
<td>
. That’s something the actual view isn’t doing yet, so
we should see a test failure:
self.assertEqual(response.content.decode(), expected_html)
AssertionError: 'A new list item' != '<html>\n <head>\n [...]
Good, our deliberately silly return value is now no longer fooling our tests, so we are
allowed to rewrite our view, and tell it to pass the POST parameter to the template:
lists/views.py (ch05l009).
def
home_page
(
request
):
return
render
(
request
,
'home.html'
, {
'new_item_text'
:
request
.
POST
[
'item_text'
],
})
Running the unit tests again:
ERROR: test_home_page_returns_correct_html (lists.tests.HomePageTest)
[...]
'new_item_text': request.POST['item_text'],
KeyError: 'item_text'
An
unexpected failure
.
If you remember the rules for reading tracebacks, you’ll spot that it’s actually a failure
in a
different
test. We got the actual test we were working on to pass, but the unit tests
have picked up an unexpected consequence, a regression: we broke the code path where
there is no POST request.
56
|
Chapter 5: Saving User Input