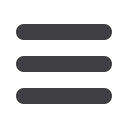

Some people like to make their unit tests into a tests folder straight
away, as soon as they start a project, with the addition of another file,
test_forms.py
. That’s a perfectly good idea; I just thought I’d wait un‐
til it became necessary, to avoid doing too much housekeeping all in
the first chapter!
Unit Testing Model Validation and the self.assertRaises Context
Manager
Let’s add a new test method to
ListAndItemModelsTest
, which tries to create a blank
list item:
lists/tests/test_models.py (ch10l012-1).
from
django.core.exceptions
import
ValidationError
[
...
]
class
ListAndItemModelsTest
(
TestCase
):
[
...
]
def
test_cannot_save_empty_list_items
(
self
):
list_
=
List
.
objects
.
create
()
item
=
Item
(
list
=
list_
,
text
=
''
)
with
self
.
assertRaises
(
ValidationError
):
item
.
save
()
If you’re new to Python, you may never have seen the
with
state‐
ment. It’s used with what are called “context managers”, which wrap a
block of code, usually with some kind of setup, cleanup, or error-
handling code. There’s a good write-up in the
Python 2.5 release notes.
This is a new unit testing technique: when we want to check that doing something will
raise an error, we can use the
self.assertRaises
context manager. We could have used
something like this instead:
try
:
item
.
save
()
self
.
fail
(
'The save should have raised an exception'
)
except
ValidationError
:
pass
But the
with
formulation is neater. Now, we can try running the test, and see it fail:
item.save()
AssertionError: ValidationError not raised
Using Model-Layer Validation
|
177