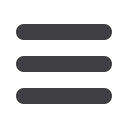

•
Remove hardcoded URLs from views.py
Now the model validation raises an exception, which comes up through our view:
[...]
File "/workspace/superlists/lists/views.py", line 11, in new_list
item.full_clean()
[...]
django.core.exceptions.ValidationError: {'text': ['This field cannot be
blank.']}
So we try our first approach: using a
try/except
to detect errors. Obeying the Testing
Goat, we start with just the
try/except
and nothing else. The tests should tell us what
to code next…
lists/views.py (ch10l015).
from
django.core.exceptions
import
ValidationError
[
...
]
def
new_list
(
request
):
list_
=
List
.
objects
.
create
()
item
=
Item
.
objects
.
create
(
text
=
request
.
POST
[
'item_text'
],
list
=
list_
)
try
:
item
.
full_clean
()
except
ValidationError
:
pass
return
redirect
(
'/lists/
%d
/'
%
(
list_
.
id
,))
That gets us back to the 302 != 200:
AssertionError: 302 != 200
Let’s return a rendered template then, which should take care of the template check as
well:
lists/views.py (ch10l016).
except
ValidationError
:
return
render
(
request
,
'home.html'
)
And the tests now tell us to put the error message into the template:
AssertionError: False is not true : Couldn't find 'You can't have an empty list
item' in response
180
|
Chapter 10: Input Validation and Test Organisation