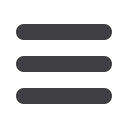

We do that by passing a new template variable in:
lists/views.py (ch10l017).
except
ValidationError
:
error
=
"You can't have an empty list item"
return
render
(
request
,
'home.html'
, {
"error"
:
error
})
Hmm, it looks like that didn’t quite work:
AssertionError: False is not true : Couldn't find 'You can't have an empty list
item' in response
A little print-based debug…
lists/tests/test_views.py.
expected_error
=
"You can't have an empty list item"
(
response
.
content
.
decode
())
self
.
assertContains
(
response
,
expected_error
)
…will show us the cause: Django has
HTML-escapedthe apostrophe:
[...]
<span class="help-block">You can't have an
empty list item</span>
We could hack something like this into our test:
expected_error
=
"You can't have an empty list item"
But using Django’s helper function is probably a better idea:
lists/tests/test_views.py (ch10l019).
from
django.utils.html
import
escape
[
...
]
expected_error
=
escape
(
"You can't have an empty list item"
)
self
.
assertContains
(
response
,
expected_error
)
That passes!
Ran 12 tests in 0.047s
OK
Checking Invalid Input Isn’t Saved to the Database
Before we go further though, did you notice a little logic error we’ve allowed to creep
into our implementation? We’re currently creating an object, even if validation fails:
lists/views.py.
item
=
Item
.
objects
.
create
(
text
=
request
.
POST
[
'item_text'
],
list
=
list_
)
try
:
item
.
full_clean
()
except
ValidationError
:
[
...
]
Let’s add a new unit test to make sure that empty list items don’t get saved:
Surfacing Model Validation Errors in the View
|
181