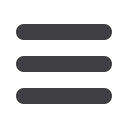

So did I break the rule about never refactoring against failing tests?
In this case, it’s allowed, because the refactor is required to get our
new functionality to work. You should definitely never refactor
against failing
unit
tests. But in my book it’s OK for the FT for the
current story you’re working on to be failing. If you prefer a clean test
run, you could add a skip to the current FT.
Enforcing Model Validation in view_list
We still want the addition of items to existing lists to be subject to our model validation
rules. Let’s write a new unit test for that; it’s very similar to the one for the home page,
with just a couple of tweaks:
lists/tests/test_views.py (ch10l024).
class
ListViewTest
(
TestCase
):
[
...
]
def
test_validation_errors_end_up_on_lists_page
(
self
):
list_
=
List
.
objects
.
create
()
response
=
self
.
client
.
post
(
'/lists/
%d
/'
%
(
list_
.
id
,),
data
=
{
'item_text'
:
''
}
)
self
.
assertEqual
(
response
.
status_code
,
200
)
self
.
assertTemplateUsed
(
response
,
'list.html'
)
expected_error
=
escape
(
"You can't have an empty list item"
)
self
.
assertContains
(
response
,
expected_error
)
That should fail, because our viewcurrently does not do any validation, and just redirects
for all POSTs:
self.assertEqual(response.status_code, 200)
AssertionError: 302 != 200
Here’s an implementation:
lists/views.py (ch10l025).
def
view_list
(
request
,
list_id
):
list_
=
List
.
objects
.
get
(
id
=
list_id
)
error
=
None
if
request
.
method
==
'POST'
:
try
:
item
=
Item
(
text
=
request
.
POST
[
'item_text'
],
list
=
list_
)
item
.
full_clean
()
item
.
save
()
return
redirect
(
'/lists/
%d
/'
%
(
list_
.
id
,))
except
ValidationError
:
error
=
"You can't have an empty list item"
return
render
(
request
,
'list.html'
, {
'list'
:
list_
,
'error'
:
error
})
186
|
Chapter 10: Input Validation and Test Organisation