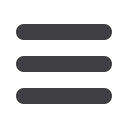

lists/views.py (ch10l026-3).
def
new_list
(
request
):
[
...
]
return
redirect
(
'view_list'
,
list_
.
id
)
That would get the unit and functional tests passing, but the
redirect
function can do
even better magic than that! In Django, because model objects are often associated with
a particular URL, you can define a special function called
get_absolute_url
which says
what page displays the item. It’s useful in this case, but it’s also useful in the Django
admin (which I don’t cover in the book, but you’ll soon discover for yourself): it will let
you jump from looking at an object in the admin view to looking at the object on the
live site. I’d always recommend defining a
get_absolute_url
for a model whenever
there is one that makes sense; it takes no time at all.
All it takes is a super-simple unit test in
test_models.py
:
lists/tests/test_models.py (ch10l026-4).
def
test_get_absolute_url
(
self
):
list_
=
List
.
objects
.
create
()
self
.
assertEqual
(
list_
.
get_absolute_url
(),
'/lists/
%d
/'
%
(
list_
.
id
,))
Which gives:
AttributeError: 'List' object has no attribute 'get_absolute_url'
And the implementation is to use Django’s
reverse
function, which essentially does the
reverse of what Django normally does with
urls.py
(see
docs ):
lists/models.py (ch10l026-5).
from
django.core.urlresolvers
import
reverse
class
List
(
models
.
Model
):
def
get_absolute_url
(
self
):
return
reverse
(
'view_list'
,
args
=
[
self
.
id
])
And now we can use it in the view—the
redirect
function just takes the object we want
to redirect to, and it uses
get_absolute_url
under the hood automagically!
lists/views.py (ch10l026-6).
def
new_list
(
request
):
[
...
]
return
redirect
(
list_
)
There’s more info in the
Django docs. Quick check that the unit tests still pass:
OK
Then we do the same to
view_list
:
Refactor: Removing Hardcoded URLs
|
189