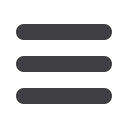

Exploring the Forms API with a Unit Test
Let’s do a little experimenting with forms by using a unit test. My plan is to iterate
towards a complete solution, and hopefully introduce forms gradually enough that
they’ll make sense if you’ve never seen them before.
First we add a new file for our form unit tests, and we start with a test that just looks at
the form HTML:
lists/tests/test_forms.py.
from
django.test
import
TestCase
from
lists.forms
import
ItemForm
class
ItemFormTest
(
TestCase
):
def
test_form_renders_item_text_input
(
self
):
form
=
ItemForm
()
self
.
fail
(
form
.
as_p
())
form.as_p()
renders the form as HTML. This unit test is using a
self.fail
for some
exploratory coding. You could just as easily use a
manage.py shell
session, although
you’d need to keep reloading your code for each change.
Let’s make a minimal form. It inherits from the base
Form
class, and has a single field
called
item_text
:
lists/forms.py.
from
django
import
forms
class
ItemForm
(
forms
.
Form
):
item_text
=
forms
.
CharField
()
We now see a failure message which tells us what the auto-generated form HTML will
look like:
self.fail(form.as_p())
AssertionError: <p><label for="id_item_text">Item text:</label> <input
id="id_item_text" name="item_text" type="text" /></p>
It’s already pretty close to what we have in
base.html
. We’re missing the placeholder
attribute and the Bootstrap CSS classes. Let’s make our unit test into a test for that:
lists/tests/test_forms.py.
class
ItemFormTest
(
TestCase
):
def
test_form_item_input_has_placeholder_and_css_classes
(
self
):
form
=
ItemForm
()
self
.
assertIn
(
'placeholder="Enter a to-do item"'
,
form
.
as_p
())
self
.
assertIn
(
'class="form-control input-lg"'
,
form
.
as_p
())
That gives us a fail which justifies some real coding. How can we customise the input
for a form field? Using a “widget”. Here it is with just the placeholder:
194
|
Chapter 11: A Simple Form