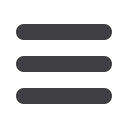

lists/tests/test_views.py (ch11l013).
from
lists.forms
import
ItemForm
class
HomePageTest
(
TestCase
):
def
test_root_url_resolves_to_home_page_view
(
self
):
[
...
]
def
test_home_page_returns_correct_html
(
self
):
request
=
HttpRequest
()
[
...
]
def
test_home_page_renders_home_template
(
self
):
response
=
self
.
client
.
get
(
'/'
)
self
.
assertTemplateUsed
(
response
,
'home.html'
)
#
def
test_home_page_uses_item_form
(
self
):
response
=
self
.
client
.
get
(
'/'
)
self
.
assertIsInstance
(
response
.
context
[
'form'
],
ItemForm
)
#
We’ll use the helper method
assertTemplateUsed
to replace our old manual test
of the template.
We use
assertIsInstance
to check that our view uses the right kind of form.
That gives us:
KeyError: 'form'
So we use the form in our home page view:
lists/views.py (ch11l014).
[
...
]
from
lists.forms
import
ItemForm
from
lists.models
import
Item
,
List
def
home_page
(
request
):
return
render
(
request
,
'home.html'
, {
'form'
:
ItemForm
()})
OK, now let’s try using it in the template—we replace the old
<input ..>
with
{{ form.text }}
:
lists/templates/base.html (ch11l015).
<form
method=
"POST"
action=
"{% block form_action %}{% endblock %}"
>
{{ form.text }}
{% csrf_token %}
{% if error %}
<div
class=
"form-group has-error"
>
{{ form.text }}
renders just the HTML input for the
text
field of the form.
Now the old test is out of date:
Using the Form in Our Views
|
199