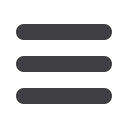

lists/views.py.
except
ValidationError
:
error
=
"You can't have an empty list item"
return
render
(
request
,
'home.html'
, {
"error"
:
error
})
We’ll want to use the form in this view too. Before we make any more changes though,
let’s do a commit:
$
git status
$
git commit -am"rename all item input ids and names. still broken"
Using the Form in a View That Takes POST Requests
Now we want to adjust the unit tests for the
new_list
view, especially the one that deals
with validation. Let’s take a look at it now:
lists/tests/test_views.py.
class
NewListTest
(
TestCase
):
[
...
]
def
test_validation_errors_are_sent_back_to_home_page_template
(
self
):
response
=
self
.
client
.
post
(
'/lists/new'
,
data
=
{
'text'
:
''
})
self
.
assertEqual
(
response
.
status_code
,
200
)
self
.
assertTemplateUsed
(
response
,
'home.html'
)
expected_error
=
escape
(
"You can't have an empty list item"
)
self
.
assertContains
(
response
,
expected_error
)
Adapting the Unit Tests for the new_list View
For a start this test is testing too many things at once, so we’ve got an opportunity to
clarify things here. We should split out two different assertions:
• If there’s a validation error, we should render the home template, with a 200.
• If there’s a validation error, the response should contain our error text.
And we can add a new one too:
• If there’s a validation error, we should pass our form object to the template.
And while we’re at it, we’ll use a constant instead of a hardcoded string for that error
message:
lists/tests/test_views.py (ch11l023).
from
lists.forms
import
ItemForm
,
EMPTY_LIST_ERROR
[
...
]
class
NewListTest
(
TestCase
):
[
...
]
def
test_for_invalid_input_renders_home_template
(
self
):
response
=
self
.
client
.
post
(
'/lists/new'
,
data
=
{
'text'
:
''
})
Using the Form in a View That Takes POST Requests
|
203