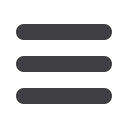

form
=
ItemForm
(
data
=
{
'text'
:
'do me'
})
new_item
=
form
.
save
(
for_list
=
list_
)
self
.
assertEqual
(
new_item
,
Item
.
objects
.
first
())
self
.
assertEqual
(
new_item
.
text
,
'do me'
)
self
.
assertEqual
(
new_item
.
list
,
list_
)
We then make sure that the item is correctly saved to the database, with the right at‐
tributes:
TypeError: save() got an unexpected keyword argument 'for_list'
And here’s how we can implement our custom save method:
lists/forms.py (ch11l034).
def
save
(
self
,
for_list
):
self
.
instance
.
list
=
for_list
return
super
()
.
save
()
The
.instance
attribute on a form represents the database object that is being modified
or created. And I only learned that as I was writing this chapter! There are other ways
of getting this to work, including manually creating the object yourself, or using the
commit=False
argument to save, but this is the neatest I think. We’ll explore a different
way of making a form “know” what list it’s for in the next chapter:
Ran 24 tests in 0.086s
OK
Finally we can refactor our views.
new_list
first:
lists/views.py.
def
new_list
(
request
):
form
=
ItemForm
(
data
=
request
.
POST
)
if
form
.
is_valid
():
list_
=
List
.
objects
.
create
()
form
.
save
(
for_list
=
list_
)
return
redirect
(
list_
)
else
:
return
render
(
request
,
'home.html'
, {
"form"
:
form
})
Rerun the test to check everything still passes:
Ran 24 tests in 0.086s
OK
And now
view_list
:
lists/views.py.
def
view_list
(
request
,
list_id
):
list_
=
List
.
objects
.
get
(
id
=
list_id
)
form
=
ItemForm
()
if
request
.
method
==
'POST'
:
form
=
ItemForm
(
data
=
request
.
POST
)
if
form
.
is_valid
():
form
.
save
(
for_list
=
list_
)
Using the Form’s Own Save Method
|
209