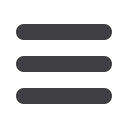

test_CAN_save_same_item_to_different_lists
item.full_clean() # should not raise
[...]
django.core.exceptions.ValidationError: {'text': ['Item with this Text already
exists.']}
An Aside on When to Test for Developer Stupidity
One of the judgement calls in testing is when you should write tests that sound like
“check we haven’t done something stupid”. In general, you should be wary of these.
In this case, we’ve written a test to check that you can’t save duplicate items to the same
list. Now, the simplest way to get that test to pass, the way in which you’d write the least
lines of code, would be to make it impossible to save
any
duplicate items. That justifies
writing another test, despite the fact that it would be a “stupid” or “wrong” thing for us
to code.
But you can’t be writing tests for every possible way we could have coded something
wrong. If you have a function that adds two numbers, you can write a couple of tests:
assert
adder
(
1
,
1
)
==
2
assert
adder
(
2
,
1
)
==
3
But you have the right to assume that the implementation isn’t deliberately screwey or
perverse:
def
adder
(
a
,
b
):
# unlikely code!
if
a
==
3
:
return
666
else
:
return
a
+
b
One way of putting it is that you should trust yourself not to do something
deliberate‐
ly
stupid, but not something
accidentally
stupid.
Just like
ModelForm
s, models have a
class Meta
, and that’s where we can implement a
constraint which says that that an item must be unique for a particular list, or in other
words, that
text
and
list
must be unique together:
lists/models.py (ch09l031).
class
Item
(
models
.
Model
):
text
=
models
.
TextField
(
default
=
''
)
list
=
models
.
ForeignKey
(
List
,
default
=
None
)
class
Meta
:
unique_together
=
(
'list'
,
'text'
)
You might want to take a quick peek at the
Django docs on model Meta attributesat this
point.
Another FT for Duplicate Items
|
213