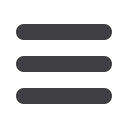

$
python3 manage.py test functional_tests.test_list_item_validation
[...]
selenium.common.exceptions.NoSuchElementException: Message: 'Unable to locate
element: {"method":"css selector","selector":".has-error"}' ; Stacktrace:
Ran 2 tests in 9.613s
OK, so we know the first of the two tests passes now. Is there a way to run just the failing
one, I hear you ask? Why yes indeed:
$
python3 manage.py test functional_tests.\
test_list_item_validation.ItemValidationTest.test_cannot_add_duplicate_items
[...]
selenium.common.exceptions.NoSuchElementException: Message: 'Unable to locate
element: {"method":"css selector","selector":".has-error"}' ; Stacktrace:
Preventing Duplicates at the Model Layer
Here’s what we really wanted to do. It’s a new test that checks that duplicate items in the
same list raise an error:
lists/tests/test_models.py (ch09l028).
def
test_duplicate_items_are_invalid
(
self
):
list_
=
List
.
objects
.
create
()
Item
.
objects
.
create
(
list
=
list_
,
text
=
'bla'
)
with
self
.
assertRaises
(
ValidationError
):
item
=
Item
(
list
=
list_
,
text
=
'bla'
)
item
.
full_clean
()
And, while it occurs to us, we add another test to make sure we don’t overdo it on our
integrity constraints:
lists/tests/test_models.py (ch09l029).
def
test_CAN_save_same_item_to_different_lists
(
self
):
list1
=
List
.
objects
.
create
()
list2
=
List
.
objects
.
create
()
Item
.
objects
.
create
(
list
=
list1
,
text
=
'bla'
)
item
=
Item
(
list
=
list2
,
text
=
'bla'
)
item
.
full_clean
()
# should not raise
I always like to put a little comment for tests which are checking that a particular use
case should
not
raise an error; otherwise it can be hard to see what’s being tested.
AssertionError: ValidationError not raised
If we want to get it deliberately wrong, we can do this:
lists/models.py (ch09l030).
class
Item
(
models
.
Model
):
text
=
models
.
TextField
(
default
=
''
,
unique
=
True
)
list
=
models
.
ForeignKey
(
List
,
default
=
None
)
That lets us check that our second test really does pick up on this problem:
Traceback (most recent call last):
File "/workspace/superlists/lists/tests/test_models.py", line 62, in
212
|
Chapter 12: More Advanced Forms