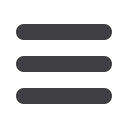

def
test_for_invalid_input_shows_error_on_page
(
self
):
response
=
self
.
post_invalid_input
()
self
.
assertContains
(
response
,
escape
(
EMPTY_LIST_ERROR
))
By making a little helper function,
post_invalid_input
, we can make four separate
tests without duplicating lots of lines of code.
We’ve seen this several times now. It often feels more natural to write view tests as a
single, monolithic block of assertions—the view should do this and this and this then
return that with this. But breaking things out intomultiple tests is definitely worthwhile;
as we saw in previous chapters, it helps you isolate the exact problem you may have,
when you later come and change your code and accidentally introduce a bug. Helper
methods are one of the tools that lower the psychological barrier.
For example, now we can see there’s just one failure, and it’s a clear one:
FAIL: test_for_invalid_input_shows_error_on_page
(lists.tests.test_views.ListViewTest)
AssertionError: False is not true : Couldn't find 'You can't have an empty
list item' in response
Now let’s see if we can properly rewrite the view to use our form. Here’s a first cut:
lists/views.py.
def
view_list
(
request
,
list_id
):
list_
=
List
.
objects
.
get
(
id
=
list_id
)
form
=
ItemForm
()
if
request
.
method
==
'POST'
:
form
=
ItemForm
(
data
=
request
.
POST
)
if
form
.
is_valid
():
Item
.
objects
.
create
(
text
=
request
.
POST
[
'text'
],
list
=
list_
)
return
redirect
(
list_
)
return
render
(
request
,
'list.html'
, {
'list'
:
list_
,
"form"
:
form
})
That gets the unit tests passing:
Ran 23 tests in 0.086s
OK
How about the FTs?
$
python3 manage.py test functional_tests
Creating test database for alias 'default'...
...
---------------------------------------------------------------------
Ran 3 tests in 12.154s
OK
Destroying test database for alias 'default'...
Woohoo! Can you feel that feeling of relief wash over you? We’ve just made a major
change to our small app—that input field, with its name and ID, is absolutely critical to
making everything work. We’ve touched seven or eight different files, doing a refactor
Using the Form in the Other View
|
207