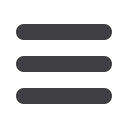

self
.
assertEqual
(
response
.
status_code
,
200
)
self
.
assertTemplateUsed
(
response
,
'home.html'
)
def
test_validation_errors_are_shown_on_home_page
(
self
):
response
=
self
.
client
.
post
(
'/lists/new'
,
data
=
{
'text'
:
''
})
self
.
assertContains
(
response
,
escape
(
EMPTY_LIST_ERROR
))
def
test_for_invalid_input_passes_form_to_template
(
self
):
response
=
self
.
client
.
post
(
'/lists/new'
,
data
=
{
'text'
:
''
})
self
.
assertIsInstance
(
response
.
context
[
'form'
],
ItemForm
)
Much better. Each test is now clearly testing one thing, and, with a bit of luck, just one
will fail and tell us what to do:
$
python3 manage.py test lists
[...]
======================================================================
ERROR: test_for_invalid_input_passes_form_to_template
(lists.tests.test_views.NewListTest)
---------------------------------------------------------------------
Traceback (most recent call last):
File "/workspace/superlists/lists/tests/test_views.py", line 55, in
test_for_invalid_input_passes_form_to_template
self.assertIsInstance(response.context['form'], ItemForm)
[...]
KeyError: 'form'
---------------------------------------------------------------------
Ran 19 tests in 0.041s
FAILED (errors=1)
Using the Form in the View
And here’s how we use the form in the view:
lists/views.py.
def
new_list
(
request
):
form
=
ItemForm
(
data
=
request
.
POST
)
#
if
form
.
is_valid
():
#
list_
=
List
.
objects
.
create
()
Item
.
objects
.
create
(
text
=
request
.
POST
[
'text'
],
list
=
list_
)
return
redirect
(
list_
)
else
:
return
render
(
request
,
'home.html'
, {
"form"
:
form
})
#
We pass the
request.POST
data into the form’s constructor.
We use
form.is_valid()
to determine whether this is a good or a bad
submission.
204
|
Chapter 11: A Simple Form