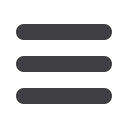

AssertionError: [<Item: Item object>, <Item: Item object>, <Item: Item object>]
!= [<Item: Item object>, <Item: Item object>, <Item: Item object>]
We need a better string representation for our objects. Let’s add another unit test:
Ordinarily you would be wary of adding more failing tests when you
already have some—it makes reading test output that much more
complicated, and just generally makes you nervous. Will we ever get
back to a working state? In this case, they’re all quite simple tests, so
I’m not worried.
lists/tests/test_models.py (ch12l008).
def
test_string_representation
(
self
):
item
=
Item
(
text
=
'some text'
)
self
.
assertEqual
(
str
(
item
),
'some text'
)
That gives us:
AssertionError: 'Item object' != 'some text'
As well as the other two failures. Let’s start fixing them all now:
lists/models.py (ch09l034).
class
Item
(
models
.
Model
):
[
...
]
def
__str__
(
self
):
return
self
.
text
in Python 2.x versions of Django, the string representation method
used to be
__unicode__
. Like much string handling, this is simpli‐
fied in Python 3. See the
docs.
Now we’re down to two failures, and the ordering test has a more readable failure
message:
AssertionError: [<Item: 3>, <Item: i1>, <Item: item 2>] != [<Item: i1>, <Item:
item 2>, <Item: 3>]
We can fix that in the
class Meta
:
lists/models.py (ch09l035).
class
Meta
:
ordering
=
(
'id'
,)
unique_together
=
(
'list'
,
'text'
)
Does that work?
AssertionError: [<Item: i1>, <Item: item 2>, <Item: 3>] != [<Item: i1>, <Item:
item 2>, <Item: 3>]
Another FT for Duplicate Items
|
215